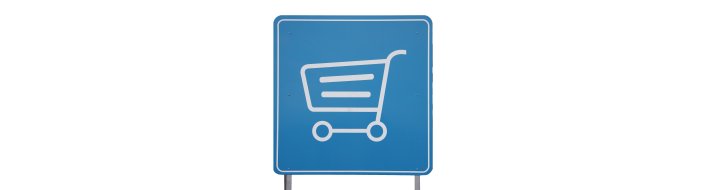
While working on the online store of D-Sight, it was necessary to include a link back to the cart when a user is on the checkout page. It is possible to click on the Cancel button but this is a bit counter-intuitive and one might not know whether the cart will remain or whether it will be emptied. Therefore, two options have been considered:
- Change the Cancel button label to rename it “Back to cart” for instance
- Modify the cart pane in the checkout view to include additional information (such as a “Back to cart” link)
Change Ubercart Button Labels (not recommended)
The first option was the easiest to put in place. Creating a module with a hook_form_alter
would do the job and allow to rename the button with something like:
1
2
3
4
5
6
7
8
9
10
11
12
<?php
function uc_alt_cart_display_form_alter(&$form, $form_state, $form_id) {
switch ($form_id) {
case 'uc_cart_checkout_form':
$form['cancel'] = array(
'#type' => 'submit',
'#value' => 'Back to cart',
);
break;
}
}
?>
However, this solution is unfortunately not so easy to put in place as some operations are based on the button name in the cart module (uc_cart.pages.inc
) such as for instance:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
<?php
function uc_cart_checkout_form() {
global $user;
// Cancel an order when a customer clicks the 'Cancel' button.
if ($_POST['op'] == t('Cancel')) {
if (intval($_SESSION['cart_order']) > 0) {
uc_order_comment_save($_SESSION['cart_order'], 0, t('Customer cancelled this order from the checkout form.'));
unset($_SESSION['cart_order']);
}
drupal_goto('cart');
}
<some more code here>
}
?>
Implement Alternative Cart Pane
Based on this, we then had to go for the second solution which consist in implementing an alternative cart pane in the checkout page. A new module is deployed for this occasion and a special two columns template is used for the checkout page to display the alternative cart in place of the normal cart if the option is activated. The module is divided in three parts:
- The info file: classic module description
- The admin.inc file: admin interface and options
- The module file: the
authorization
,hook_menu
,hook_checkout_pane
andhook_form_alter
The admin interface is quite simple and simply allows the user to determine if the alternative cart pane should be used in the checkout page and in such case, what additional text should appear under the cart.
The corresponding code for the admin interface is displayed below.
File uc_alt_cart_display.admin.inc
:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
<?php
function uc_alt_cart_display_form(&$form_state){
$form = array();
$form['checkout'] = array(
'#type' => 'fieldset',
'#title' => t('Checkout Page'),
'#collapsible' => FALSE,
);
$form['checkout']['uc_alt_cart_use_alternative_cart'] = array(
'#type' => 'checkbox',
'#title' => t('Use alternative cart in checkout page'),
'#default_value' => variable_get('uc_alt_cart_use_alternative_cart', 1),
'#description' => t("Specify if the alternative cart should be used in the checkout page. If not selected, options below will be discarded."),
);
$form['checkout']['uc_alt_cart_additional_text'] = array(
'#type' => 'textarea',
'#title' => t('Additional text below cart'),
'#default_value' => variable_get('uc_alt_cart_additional_text', ''),
'#resizable' => TRUE,
'#description' => t("Specify the additional text that needs to be displayed below the cart summary on the checkout page"),
'#required' => FALSE,
);
return system_settings_form($form);
}
?>
File uc_alt_cart_display.module
:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
<?php
function uc_alt_cart_display_checkout_pane() {
if(variable_get('uc_alt_cart_use_alternative_cart', 1)){
// Define the alternative cart pane. This comes from the function uc_cart_checkout_pane() in the uc_cart.module
$panes[] = array(
'id' => 'alt_cart',
'callback' => 'uc_alt_cart_display_checkout_pane_uc_alt_cart_display_cart',
'title' => t('Cart contents'),
'desc' => t("Display the contents of a customer's shopping cart."),
'weight' => 0,
'process' => TRUE,
'collapsible' => FALSE,
);
return $panes;
}
}
function uc_alt_cart_display_checkout_pane_uc_alt_cart_display_cart($op, &$arg1, $arg2) {
require_once(drupal_get_path('module', 'uc_cart') . '/uc_cart_checkout_pane.inc');
switch ($op) {
case 'view':
// Get the content from the official uc_checkout_pane_cart function.
$temp_contents = uc_checkout_pane_cart($op, $arg1, $arg2);
$contents = array('contents' => array());
foreach($temp_contents['contents'] as $key => $value) {
$contents['contents']['uc_alt_cart_display_'. $key] = $value;
}
$contents['contents']['uc_alt_cart_display_cart_link'] = array(
'#value' => variable_get('uc_alt_cart_additional_text', ''),
'#weight' => "10",
);
return array('contents' => $contents, 'next-button' => FALSE);
// Other cases (review, process, ...) are not considered as we are only interested in seeing this text in the checkout page
}
}
function uc_alt_cart_display_form_alter(&$form, $form_state, $form_id) {
switch ($form_id) {
case 'uc_cart_checkout_form':
if(variable_get('uc_alt_cart_use_alternative_cart', 1)){
unset($form['panes']['cart']);
}
break;
}
}
?>
I think that most of the code is self-explanatory but in a few words, the admin interface allows the user to decide whether to use the alternative cart panel and define an additional block of text that will display under the cart. In the module part, the checkout_panes hook
allows to define the alternative cart and the callback function is in charge of calling the normal cart and adding the custom text. Finally, the form_alter
hook unsets the default cart panes if the alternative cart pane should be used (in order to avoid a double display of the cart in the checkout page). In the checkout form page, it is possible to include as well the following code to render the alternative cart only if it is defined.
File uc-cart-checkout-form.tpl.php
:
1
2
3
4
5
6
7
8
<?php
if(isset($form['panes']['alt_cart'])){
$output .= drupal_render($form['panes']['alt_cart']);
}
else{
$output .= drupal_render($form['panes']['cart']);
}
?>
Download Links
References
As often, great content was available online. Here are some of the resources that I used to achieve this alternative cart display module.
For the time being, comments are managed by Disqus, a third-party library. I will eventually replace it with another solution, but the timeline is unclear. Considering the amount of data being loaded, if you would like to view comments or post a comment, click on the button below. For more information about why you see this button, take a look at the following article.